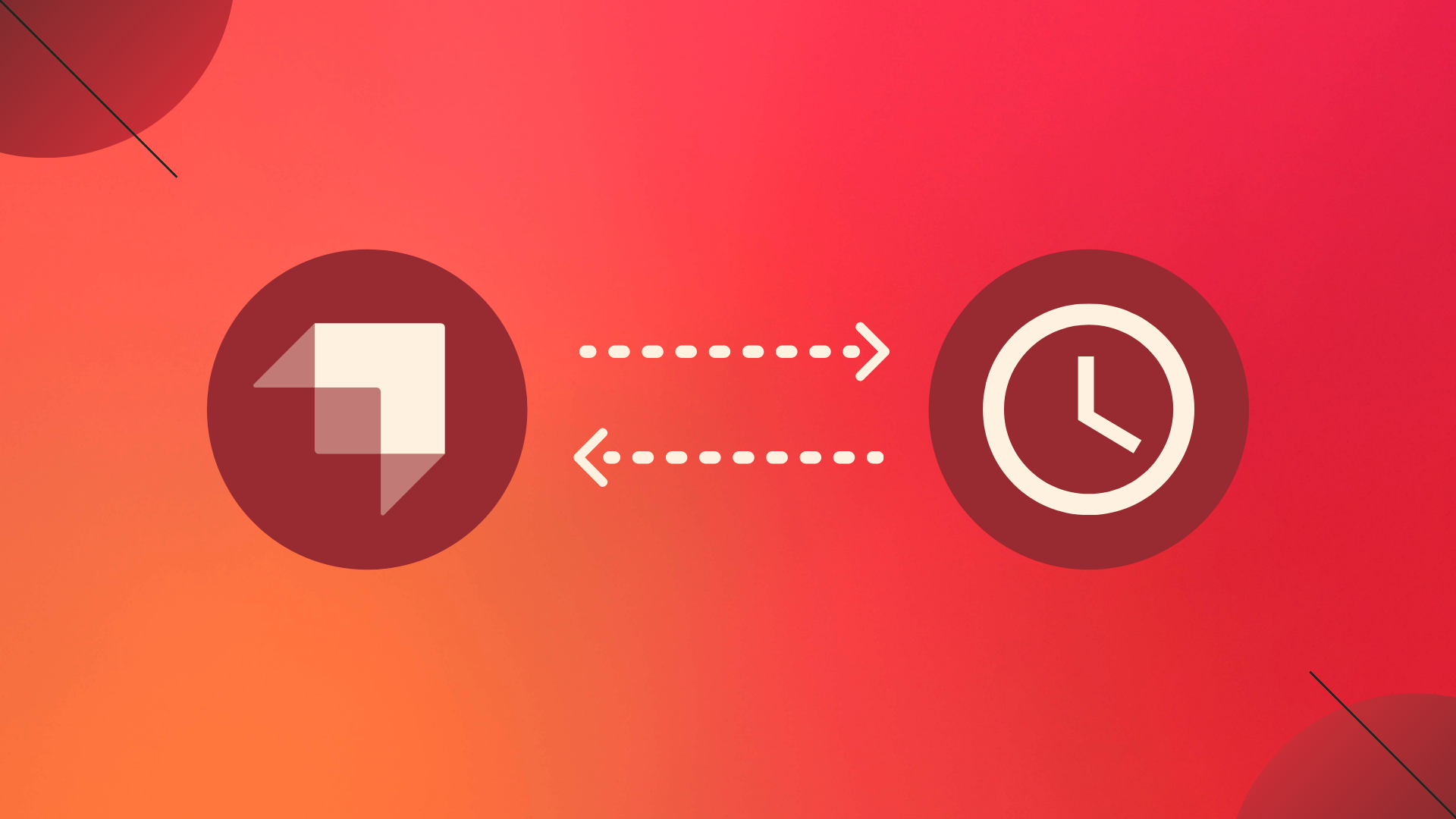
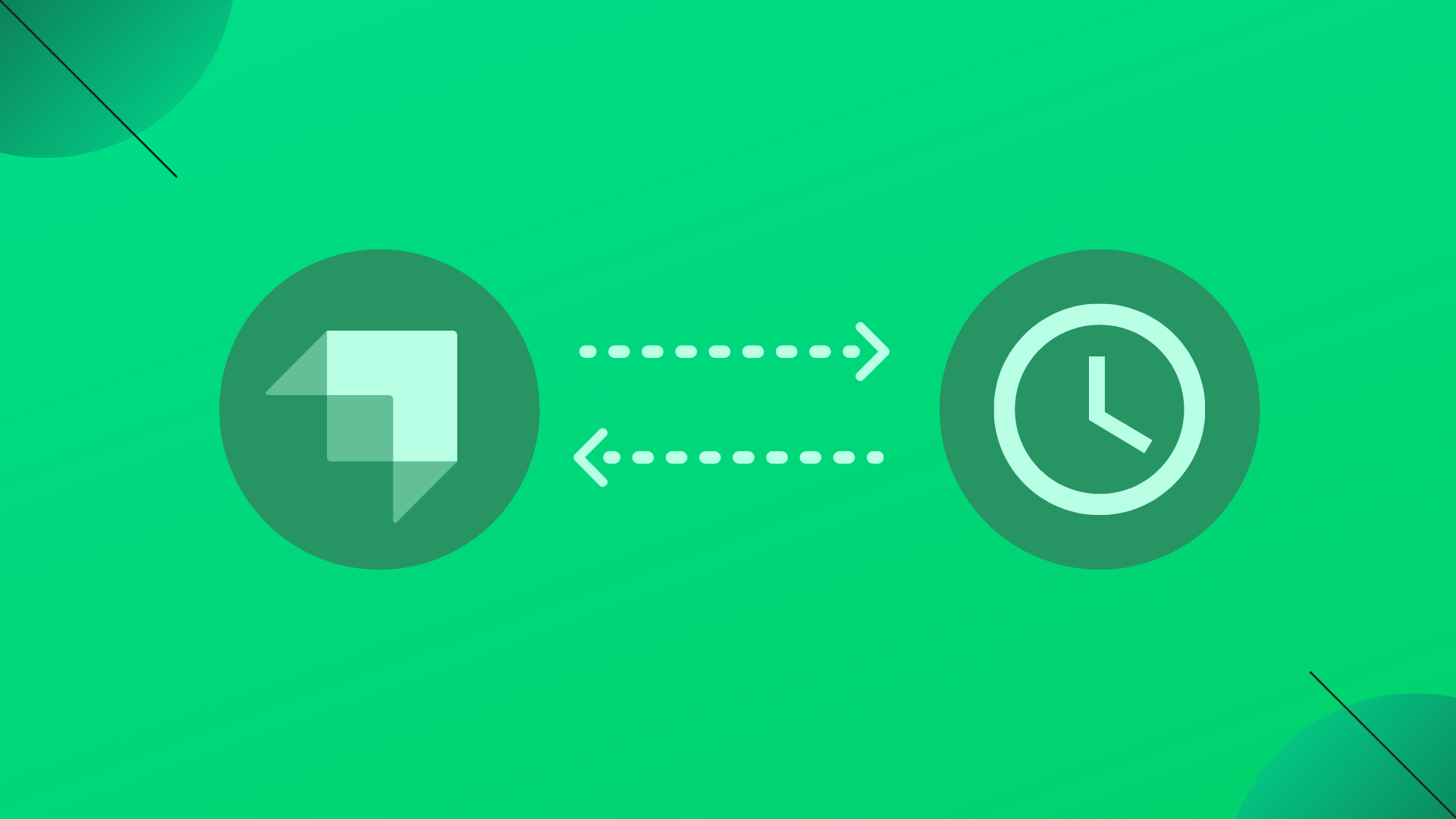
Creating a cron in Strapi for adding short links to articles
I was searching for a service to shorten my URLs for social sharing links and found short.io which offer a free plan with perfect rest API.
There can 2 approaches to add a corn job in Strapi:
- Use Strapi native cron feature (which I failed to run after multiple attempts)
- Use OS
crontab
and configurecli
based jobs outside Strapi (the approach I am using for my URL shortener cron)
-
Create a CLI command in Strapi
- Install node
short.io
sdk
yarn add short.io npm install short.io
- Add required configurations in
.env
file:
# your short.io short domain URL_SHORTENER_DOMAIN=go.adapttive.com # your short.io short domain id URL_SHORTENER_DOMAIN_ID=12345 # your short.io API key with all access URL_SHORTENER_API_KEY=xxxx # your website base URL to be shortened URL_SHORTENER_BLOG_URL=https://adapttive.com/blog/
- Create a command file in your strapi project (Ex:
/home/ubuntu/strapi/cli/shortener.js
):
#!/usr/bin/env node const strapi = require('strapi'); if (process.env.CRON_URL_SHORTENER) { strapi().load().then( async (instance) => { // fetch articles to add short URL for mongo db (refer to strapi documentation in references for MySQL query) const articles = await instance.query('article').model.find({ shortUrl: null, published_at: {$ne: null} }, ['slug', 'shortUrl']); if (articles.length > 0) { const shortio = require("short.io"); const short = new shortio(process.env.URL_SHORTENER_DOMAIN, process.env.URL_SHORTENER_DOMAIN_ID, process.env.URL_SHORTENER_API_KEY); for (const article of articles) { let link = await short.createLink({originalURL: process.env.URL_SHORTENER_BLOG_URL + article.slug}); // secureShortURL: 'https://go.adapttive.com/eMEe5R', if (link && link.secureShortURL) { console.log("slug:", article.slug); console.log("shortUrl:", link.secureShortURL); const result = await instance.query('article').model.updateOne( {_id: article.id}, {$set: {"shortUrl": link.secureShortURL}} ); } } } console.log("url added:", articles.length); // exit with 0 code instance.stop(0) } ) }
- Make
shortener.js
executable:
sudo chmod +x shortener.js
- Add the command to your Strapi project
package.json
asshortener
:
{ "name": "api-cms-adapttive-com", "private": true, "version": "0.1.0", "description": "A Strapi application", "scripts": { "develop": "strapi develop", "start": "strapi start", "build": "strapi build", "strapi": "strapi", "shortener": "node cli/shortener.js" } }
- Install node
-
Setting the CLI command incrontab
- Create log folder and file
mkdir -p /var/log/strapi touch /var/log/strapi/cron.log
- Add command in os crontab
crontab -e
- Copy and paste the below in crontab:
0 8 * * * export CRON_URL_SHORTENER=1 && cd /home/ubuntu/strapi && yarn shortener |& tee -a /var/log/strapi/cron.log